What is static variable in javascript?
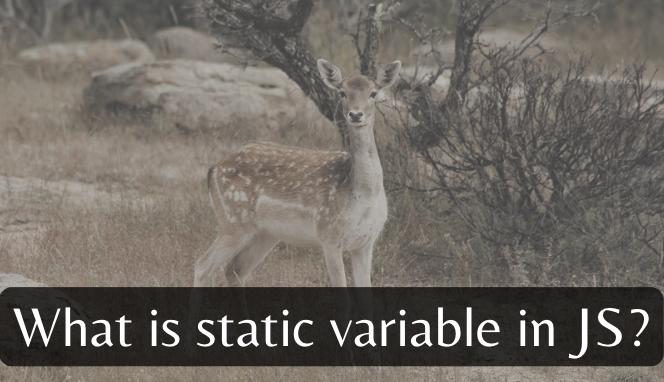
A static variable is a variable that has been allocated ‘statically’ meaning that its lifetime is the entire run of the program. These types of variables have global presence and scope. We can use static variables anywhere over the script. Unlike const variables, we can reassign and change the value of static variables.
Static variable remains in memory while the program is running, and the same value is shared and used among all the instances created in a class. Static variables, functions, properties, values can be defined by using the static keyword.
A static variable is a class property that is used in a class and not in the instance of the class. It means when accessing a static variable of class property, we do not need to create a new instance of the class by using a new keyword but can be directly accessed by getting the static instance of the class.
Why do we need static variables?
While building an application, we need certain variables, methods which need to be accessed in global scope. This is where static variables come into play where once defined a variable using a static keyword, it can be accessed throughout the application.
One of the main benefits of using static variables is it can be reassigned, changed and used across the application. Static methods can be used for creating or cloning the objects.
For example, if we have 100 employees in a company, and we need to store their details like:
- name
- address
- date_of_birth
- company_name
Then here company_name is going to be the same for all employees so here instead of duplicating company_name to all 100 employees and consuming additional space in memory, we can define company_name as static.
Syntax and using static variables
As we know now that static variables are defined using static keywords, we will define class and define some static variables, methods and use it.
class Person {
static name = 'John';
static getName() {
return `Hi! My name is ${this.name} called from the static method.`; // ${Person.name} can also be used instead of this keyword.
}
static {
console.log('Class static initialization block called');
}
}
console.log(Person.name);
// output: "John"
console.log(Person.getName());
// output: "Hi! My name is John called from the static method."
In the above example, we defined a static property, static method and static object. Whenever we run the program, the static object with console message will be initialized and called out.
So, in order to use the name property of Person class, we can simply use Person.name outside the class block. We do not need to create a new instance of object or constructor.
Similarly, in the getName function, we have used this.name to call the static name property. We can also use the class name which is Person.name to access the name property.
Difference between static, non-static and const variables
Static Variable
- Defined by using ‘static’ keyword.
- It can be reassigned and changed.
- Can be accessed using class name.
- Static methods can be creating or cloning the objects.
- Syntax: static variable_name
Non-static variable
- Defined by using ‘var’, ‘let’ keyword.
- Non-static variable is like a local variable.
- Can be accessed through the instance of class.
- Cannot be accessed inside a static method.
- Syntax: var variable_name
Const Variable
- Defined using ‘const’ keyword.
- Cannot be reassigned and changed.
- Remains the same throughout the application.
- Mostly used to declare a constant value.
- Syntax: const variable_name
Conclusion
So, the basic idea of static variables is to efficiently manage memory and keep track of the information that relates logically to an entire class. I hope you got the idea about the static variables, its uses and its importance. Happy learning!